C Programming Language Tutorial - GeeksforGeeks
- ️Mon Jun 10 2024
Last Updated : 06 Jan, 2025
C is a general-purpose, procedural, and middle-level programming language used for developing computer software, system programming, applications, games, and more. Known for its simplicity and efficiency, C is an excellent choice for beginners as it provides a strong foundation in programming concepts. C was developed by Dennis M. Ritchie at Bell Laboratories in 1972. Initially, it was created for programming the UNIX operating system.
- Bridges the gap between low-level programming (closer to hardware and machine code) and high-level programming (closer to human-readable code).
- Referred as the "mother of all programming languages" because it influenced many modern programming languages like C++, Java, Python and Go.
- C programs are platform-independent i.e. code can be compiled and run on different systems with minimal modifications.
- Does not require heavy runtime environments or libraries, making it ideal for low-resource systems.
Hello World Program
C#include <stdio.h>
int main()
{
printf("Hello World!");
return 0;
}
In this C tutorial, we’ll cover everything from basic syntax, data types, and control structures to advanced topics like pointers, memory management, and file handling. By the end, you’ll gain hands-on experience and a solid understanding of C, which is essential for mastering other programming languages like C++ and Java. Let’s dive into the world of C programming and build a strong coding foundation!
C Overview
- What is C Language?
- C Features
- C Standards
- Setting Up C Development Environment
- Hello World Program
- Compiling a C Program: Behind the Scenes
C Basics
- Tokens
- Identifiers
- Keywords
- Comments
- Variables
- Constants
- Data Types
- Data Type Modifiers
- Type Conversion
- Operators
C Input/Output
C Operators
- Arithmetic Operators
- Unary Operators
- Assignment Operators
- Logical Operators
- Bitwise Operator
- Signed Number Representation
- Operator Precedence and Associativity
C Flow Control
- if Statement
- if…else Statement
- if-else-if Ladder
- Switch Statement
- Using Range in Switch Case
- for Loop
- while looping
- do…while Loop
- continue Statement
- break Statement
- goto Statement
C Functions
- Introduction to Functions
- User-Defined Function
- Parameter Passing Techniques
- Main Function
- Inline Function
- Nested Functions
C Arrays
- Introduction to Arrays
- Length of Array
- Multidimensional Arrays
- Pass Array to Functions
- Pass a 2D Array as a Parameter
C Strings
- Introduction to Strings
- Length of String
- String Comparison
- String Copy
- String Concatenation
- Array of Strings
- String Functions
C Pointers
- Introduction to Pointers
- Pointer Arithmetics
- Pointer to Pointer (Double Pointer)
- Function Pointer
- Declare Function Pointer
- Pointer to an Array
- Constant Pointer
- Pointer vs Array
- Dangling, Void, Null and Wild Pointers
- Near, Far and Huge Pointers
- restrict Keyword
C Dynamic Memory Allocation
C Structures and Union
- Introduction to Structures
- Array of Structures
- Structure Pointer
- Structure Member Alignment
- Introduction to Unions
- Bit Fields
- Structure vs Union
- Anonymous Union and Structure
- Enumeration (or enum)
- dot (.) Operator
- typedef
C Storage Classes
- Introduction to Storage Classes
- extern Keyword
- Static Variables
- Initialization of Static Variables
- Static Functions
- Understanding “volatile” Qualifier
- Understanding the “register” Keyword
C Preprocessor
- Introduction to Preprocessors
- Preprocessor Directives
- How a Preprocessor Works?
- Header Files
- Header Files “stdio.h” vs “stdlib.h”
- Write Your Own Header File
- Macros and its Types
- Interesting Facts About Macros and Preprocessors
- # and ## Operators
- Print a Variable Name
- Multiline Macros
- Variable Length Arguments for Macros
- Branch Prediction Macros in GCC
- typedef versus #define
- Difference Between #define and const
C File Handling
- Basics of File Handling
- fopen() Function
- EOF, getc() and feof()
- fgets() and gets()
- fseek() vs rewind()
- Return Type of getchar(), fgetc() and getc()
- Read/Write Structure From/to a File
- Print Contents of File
- Delete a File
- Merge Contents of Two Files into a Third File
- printf vs sprintf vs fprintf
- getc() vs getchar() vs getch() vs getche()
C Error Handling
- Error Handling
- Using goto for Exception Handling
- Error Handling During File Operations
- Handle Divide By Zero and Multiple Exceptions
C Programs
- Basic C Programs
- Control Flow Programs
- Pattern Printing Programs
- Functions Programs
- Arrays Programs
- Strings Programs
- Conversions Programs
- Pointers Programs
- Structures and Unions Programs
- File I/O Programs
- Date and Time Programs
- More C Programs
Miscellaneous
- Date and Time
- Input-Output System Calls
- Signals
- Program Error Signals
- Socket Programming
- _Generics Keyword
- Multithreading
C Interview Questions
- Top 50 C Programming Interview Questions and Answers
- Commonly Asked C Programming Interview Questions | Set 1
- Commonly Asked C Programming Interview Questions | Set 2
- Commonly Asked C Programming Interview Questions | Set 3
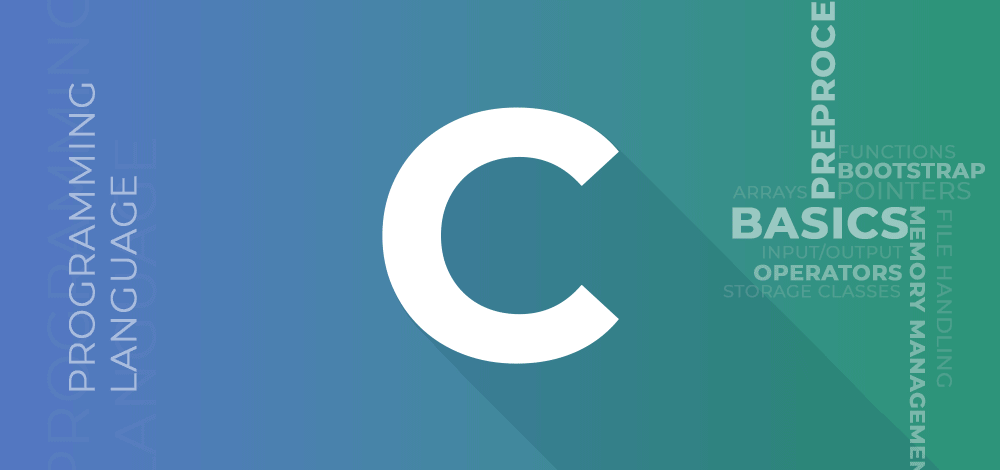
C Compiler
C compiler is a software that translates human-readable C language code into machine code or an intermediate code that can be executed by a computer’s central processing unit (CPU).
There are many C compilers available in the market, such as GNU Compiler Collection (GCC), Microsoft Visual C++ Compiler, Clang, Intel C++ Compiler, and TinyCC (TCC).
For this tutorial, we will be using the GNU-based online C compiler provided by GeeksforGeeks which is developed for beginners and is very easy to use compared to other compiler/IDE’s available on the web.
Features of C Language
There are some key features of C language that show the ability and power of C language:
- Simplicity and Efficiency: The simple syntax and structured approach make the C language easy to learn.
- Fast Speed: C is one of the fastest programming language because C is a static programming language, which is faster than dynamic languages like Javascript and Python. C is also a compiler-based which is the reason for faster code compilation and execution.
- Portable: C provides the feature that you write code once and run it anywhere on any computer. It shows the machine-independent nature of the C language.
- Memory Management: C provides lower level memory management using pointers and functions like realloc(), free(), etc.
- Pointers: C comes with pointers. Through pointers, we can directly access or interact with the memory. We can initialize a pointer as an array, variables, etc.
- Structured Language: C provides the features of structural programming that allows you to code into different parts using functions which can be stored as libraries for reusability.
Applications of C Language
C was used in programs that were used in making operating systems. C was known as a system development language because the code written in C runs as fast as the code written in assembly language.
The use of C is given below:
- Operating Systems
- Language Compilers
- Assemblers
- Text Editors
- Print Spoolers
- Network Drivers
- Modern Programs
- Databases
- Language Interpreters
- Utilities
FAQs
1. How to learn C easily?
The first steps towards learning C or any language are to write a hello world program. It gives the understanding of how to write and execute a code. After this, learn the following:
- Variables
- Operators
- Conditionals
- Loops and Errors
- Arrays and Strings
- Pointers and Memory
- Functions
- Structures
- Recursions
2. Difference between C and C++?
C | CPP |
---|---|
C is a procedural programming language. | C++ is both a procedural and object-oriented programming language. |
It does not support Function overloading. | It supports function overloading. |
Operator overloading is not supported. | Operator overloading is supported. |
C does not support data hiding which leads to security concerns. | Data hiding is supported in C++ by Data Encapsulation. |
3. Is C easy to learn for beginners?
While C is one of the easy languages, it is still a good first language choice to start with because almost all programming languages are implemented in it. It means that once you learn C language, it’ll be easy to learn more languages like C++, Java, and C#.
4. Why should we learn C first rather than C++?
C is a ‘mother of all languages.’ It provides a solid understanding of fundamental programming concepts and is considered easier to grasp. C offers versatile applications, from software development to game programming, making it an excellent choice for building a strong programming foundation.